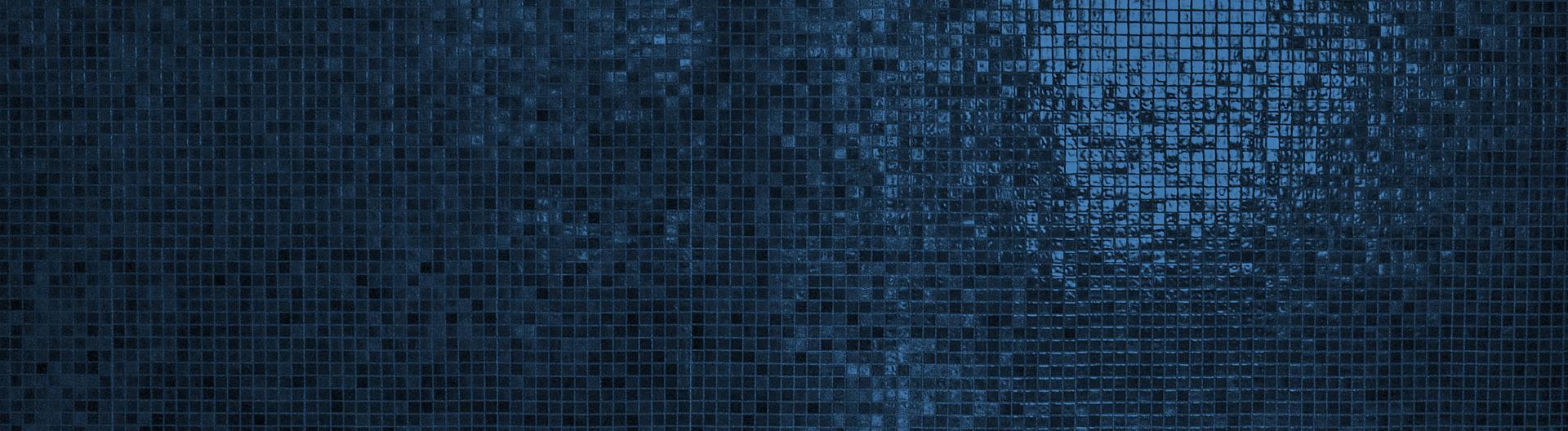
-
The Issue
Something is stretching your nice mobile layout beyond your expected 560px width. The layout displays mostly nice, but you can drag the entire site to the left and see extra space. You keep toggling open elements in the inspector trying to find the culprit lurking in its hideout plotting its next caper. You futz about with overflow: hidden. You get tricky, winnowing down widths until it all fits: "Okay, we've got 20px on each side, so you're now, uh, width: 520px." You start floating and clearing and swearing.
A possible reason is that the standard box model calculates dimensions only for elements themselves, excluding padding and borders. You might have assigned a 560px width to a container, but padding and borders push it wider as a result.
Solution
Change your box-sizing. A number of developers prefer changing the box-sizing from content-box to border-box, which includes padding and borders in width calculations. When I have 560px of space, I usually want its contents to be no more than that.
There are a couple ways to do this. The classic method is to set everything to border-box:
*, *::before, *::after { box-sizing: border-box; }
This will work nicely in just about all cases, although when you do want something to behave in the standard content-box way, you'll have to override it and all its children. This is the current standard, which is more inheritance-friendly:
html { box-sizing: border-box; } *, *:before, *:after { box-sizing: inherit; }
Other Notes
• Margin is not included, and we typically don’t want it to be. We expect margins to be "outside" an element. I do, anyway. I'm sorry if this, um, marginalizes them.
• The * selector does not include pseudo-elements by default, so if you use the first method, include them.
• This whole border-box behavior used to be "Quirks Mode" for IE6 and earlier. So, IE won that aesthetic round.
-
The Issue
So you’ve got two elements sitting atop each other, each with vertical margins. You would expect the margins to add together:
.thing { display: block; width: 100%; height: 200px; } .thing-upper { margin-bottom: 50px; } .thing-lower { margin-top: 30px; }
… so the space between them is expected to be 50 + 30 = 80 pixels. Except they’re not.
When two vertical margins meet, they usually collapse; i.e., in most cases, where the margins of two elements meet, only the larger value is preserved (or if equal, only a single value).
This is the normal flow of the box model, and there are specific cases governing this collapse (blocks with margins but no content, adjacent siblings, etc.).
Solution
Maddening before one knows what's going on, but it’s workable.
The simplest method is to stick with either top or bottom. I prefer margin-bottom, because I usually want to be consistent with how much room there is underneath something. I also often have to override a margin-top anyway (header tags, i’m looking at you).
Other Notes
• There is a -webkit-margin-after-collapse property which can be set to "separate", but of course non-Webkit browsers get no love.
• You can include an :after pseudo-element with display:table, but I like reserving my befores and afters for interesting things.
• Floats do not collapse vertical margins, but you must control width and clearance (width: 100%, clear: both).
• Negative margins are funny. If both margins are negative (who does this?), then they act as if positive, preserving the larger absolute value. If one margin is negative, the negative margin is subtracted from the positive.
• First and last child elements tend to have their vertical margins overridden by their parent, or even extend their margin outside the parent. You can muck about with the properties of the child (like its padding), which sort of "wakes it up" and allows the margins to be preserved. See MDN web docs for painstaking but succinct details.
-
Performance is foremost in this architecture and so duplication of data is nessary.
By Product ID
The following is a simple product table used to lookup products by their product_id.
cqlsh> CREATE TABLE product (product_id text, name text, description text, asset text, category text, PRIMARY KEY((product_id)));
Populate with data:
cqlsh> UPDATE product SET name = 'Hammer', description = 'For nails', category = 'Tool' WHERE product_id = 'T112233';
Select a product:
cqlsh> SELECT * FROM product WHERE product_id = 'T112244';
By Category
We duplicate our data into another table with optimized promary key and column clustering.
cqlsh> CREATE TABLE product_by_category ( product_id text, name text, description text, asset text, category text, PRIMARY KEY((category), product_id, name)) WITH CLUSTERING ORDER BY (product_id ASC, name ASC); cqlsh> UPDATE product_by_category SET description = 'For nails' WHERE product_id = 'T112233' AND category = 'Tool' AND name = 'Hammer'; cqlsh> UPDATE product_by_category SET description = 'For hammers' WHERE product_id = 'T112244' AND category = 'Hardware' AND name = 'Nails';
-
This tutorial is for mac users.
Burn Image
Insert an SD card into your computer, then open a terminal window and type:
diskutil list
You will get a list of drives. In the example below I have 3 disks, disk0, disk1 and disk2. The first disk is my hard drive and I need to be extra carefull not to accidentally overwrite it with my image. disk2 is my 8GB SD card. Two clues are (internal, physical) and *7.9 GB. See below:
I need to unmount this disk:
diskutil unmountDisk /dev/disk2
Here is an example:
Next I will use the dd command to burn my image onto the SD card:
sudo dd if=~/Desktop/some-image.img of=/dev/rdisk2 bs=5m
You can see I am using the device “rdisk2”. This is much faster than using “disk2”. I found this from a superuser.com question “Why is “/dev/rdisk” about 20 times faster than “/dev/disk” in Mac OS X”
This process will still take a good 10-20 minuts to burn an 8GB sd card. You can type “control-t” at any time to see the progress:
You should get from 4000000 to 10000000 bytes/sec (4 to 10 megabytes per second). If you get less than this you may have a bad SD card.
Once the process has finished you can insert the card into your Raspberry Pi.
Clone Image
Insert an SD card into your computer, then open a terminal window and type:
diskutil list
Again, you will use the dd command with the arguments “if” (in from file) and “of” (output to file) in reverse order from the burning example above.
sudo dd if=/dev/rdisk2 of=~/Desktop/some-image.img bs=5m
Resources
- Download Raspbian: “Raspbian is the Foundation’s official supported operating system.”
-
Install Java 8
$ sudo yum install java-1.8.0-openjdk
Add DataStax Repo for Apache Cassandra
$ sudo vi /etc/yum.repos.d/datastax.repo
Add the following lines to the new file:
[datastax-ddc] name = DataStax Repo for Apache Cassandra baseurl = http://rpm.datastax.com/datastax-ddc/3.9 enabled = 1 gpgcheck = 0
Save the file above and run:
$ sudo yum install datastax-ddc $ sudo service cassandra start $ sudo nodetool status
Add Python 2.7
$ cd /usr/src $ sudo wget http://python.org/ftp/python/2.7.6/Python-2.7.6.tgz $ sudo tar -xvzf Python-2.7.6.tgz $ cd Python-2.7.6 $ sudo ./configure --prefix=/usr/local $ sudo make $ sudo make install
Fix python libs:
$ cd /usr/lib/python2.7/ $ sudo mv site-packages/* /usr/local/lib/python2.7/site-packages/ $ sudo rm -R site-packages $ sudo ln -s /usr/local/lib/python2.7/site-packages ./
There should now be a symlink in /usr/lib/python2.7 that points site-packages to /usr/local/lib/python2.7/site-packages.
site-packages -> /usr/local/lib/python2.7/site-packages
In the /usr/local/lib/python2.7/site-packages you should see a cqlshlib folder.
Run cqlsh
# cqlsh # cqlsh> DESCRIBE keyspaces;
More Subheader
Client Work. Nothing but the good stuff: